The full source code for the key press counter is available on GitHub
Generating A Key Press Counter with Chat GPT
After a casual comment during one of my daily standups, I got the idea to write a counter program to see how many clicks I do on the computer in a given day.
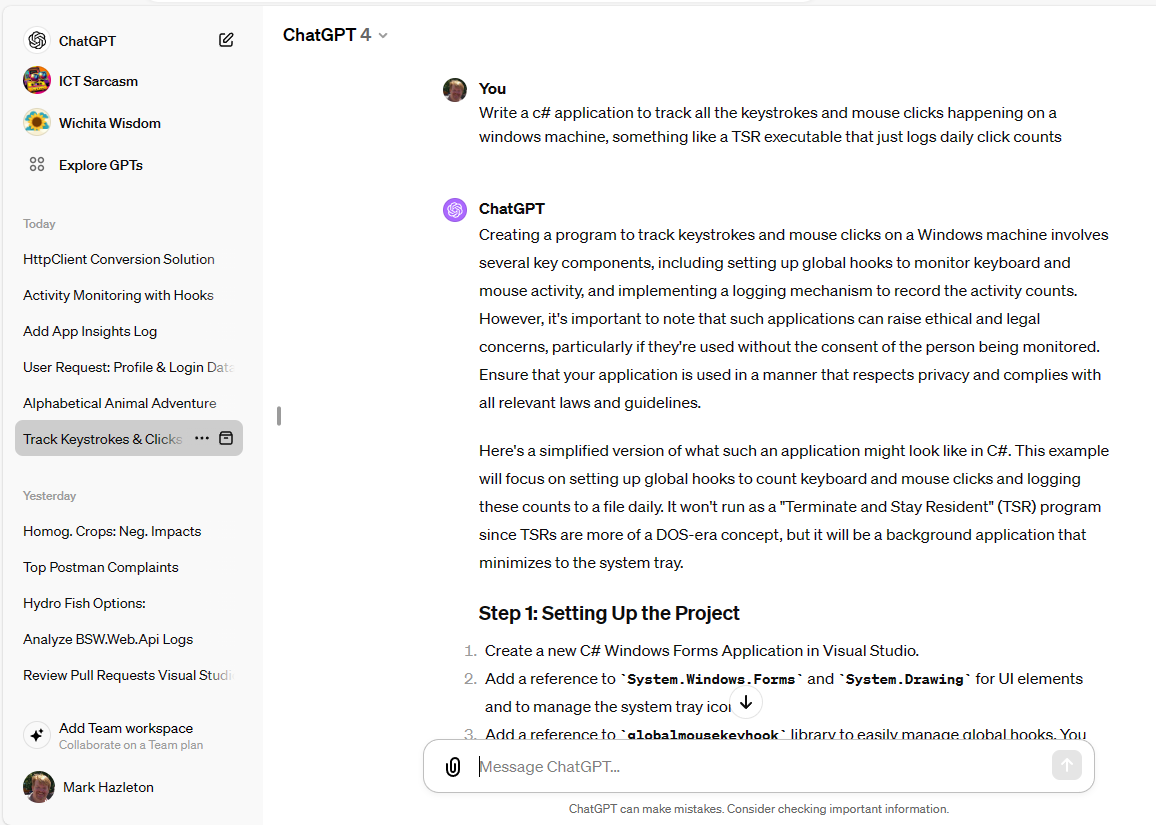
The journey into developing a utility to monitor key and mouse presses unfolded with a focus on user interaction patterns. This post delves into the ethical considerations, the technical setup, and includes snippets of the essential code.
Explore the development of a key press counter focusing on user interaction, ethical considerations, and technical insights.
Ethical Considerations and User Consent
In exploring the development of a key press counter, ChatGPT proactively raised ethical considerations, emphasizing the paramount importance of user consent and privacy.
However, it's important to note that such applications can raise ethical and legal concerns, particularly if they're used without the consent of the person being monitored. Ensure that your application is used in a manner that respects privacy and complies with all relevant laws and guidelines.
These warnings are a testament to ChatGPT's design, which is ingrained with ethical guidelines to ensure technology serves humanity responsibly. The model's ability to foreground these concerns illustrates its commitment to ethical computing, encouraging developers to prioritize transparency and adherence to privacy laws in their creations. This approach underlines the broader conversation about the responsible use of technology and the role of AI in guiding ethical decision-making in software development.
Technical Setup and Code Snippets
Application Development Steps
- Step 1: Setup Your Development Environment
- Install Visual Studio and ensure .NET Framework or .NET Core is set up. Create a new Windows Forms Application project.
- Step 2: Implement Global Hooks
- Use the `globalmousekeyhook` library to listen for system-wide keyboard and mouse events. Install it via NuGet Package Manager in Visual Studio.
- Step 3: Create Event Handlers
- Write methods to handle keyboard and mouse events. Increment counters for key presses and mouse clicks within these methods.
- Step 4: Log the Activity
- Store the counts in a file or database. Use `System.IO.File` methods for file operations, ensuring data is written periodically or upon certain triggers.
- Step 5: Build and Test
- Compile your project in Visual Studio, fix any errors, and run the application to ensure it tracks key presses and mouse clicks as expected.
- Step 6: Ensure Ethical Use
- Clearly communicate the application's functionality to users and obtain their consent before use. Consider privacy laws and ethical guidelines.
We leveraged global hooks in .NET to monitor activities. Key aspects of the implementation include setting up hooks, event handling, and logging.
// Initialize global hooks
globalHook = Hook.GlobalEvents();
globalHook.KeyPress += GlobalHookKeyPress;
globalHook.MouseClick += GlobalHookMouseClick;
// Event handler for key presses
private void GlobalHookKeyPress(object sender, KeyPressEventArgs e)
{
keyPressCount++;
}
// Event handler for mouse clicks
private void GlobalHookMouseClick(object sender, MouseEventArgs e)
{
mouseClickCount++;
}
Source Code
The full source code for the key press counter is available on GitHub. Feel free to explore and adapt it for your projects. Key Press Counter on GitHub
Reflecting on the Journey
This tool serves as a testament to balancing innovation with ethical standards, highlighting the importance of thoughtful software development.
Incorporating ChatGPT into coding projects not only adds a layer of technological interaction but also brings to light the platform's built-in measures to address ethical implications in its outputs. This aspect of our coding journey highlights the importance of ethical considerations in AI-assisted development, offering a deeper understanding of responsible AI use.
Update: Creating a Tray Icon and Balloon Notifications
After implementing the key press counter, I decided to enhance the user experience by adding a tray icon and balloon notifications on double click. This allows users to easily access the counter and receive notifications without the need to keep the application window open.
Creating the Tray Icon and Balloon Notifications
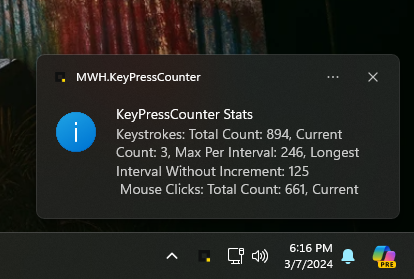
To create the tray icon, I used the `NotifyIcon` class from the `System.Windows.Forms` namespace.
To display balloon notifications on double click, I utilized the `ShowBalloonTip` method of the `NotifyIcon` class.
With these additions, users can now access the key press counter by double-clicking the tray icon and receive notifications about the counter's status.
Here's how I achieved this functionality:
trayIcon = new NotifyIcon()
{
Icon = new Icon("favicon.ico"), // Set your icon here
ContextMenuStrip = CreateContextMenu(), // Optional: Set if you want a right-click menu
Visible = true,
Text = "Double Click Icon for Stats"
};
// Optional: Handle double-click event
trayIcon.DoubleClick += TrayIcon_DoubleClick;
private void UpdateTrayIconText()
{
string log = $"\n Keystrokes: {keyPressCounter} \n Mouse Clicks: {mouseClickCounter}";
trayIcon.ShowBalloonTip(5000, "KeyPressCounter Stats", $"{log}", ToolTipIcon.Info);
}
The integration of a tray icon and balloon notifications enhances the usability of the key press counter application. It provides a convenient way for users to access the counter and stay informed about their key press activity. By leveraging ChatGPT and the capabilities of the `NotifyIcon` class, I was able to create a more user-friendly experience.